· Phani Veludurthi · Dotnet · 2 min read
Asynchronous Programming in .NET: Understanding Tasks and Async/Await
In the realm of modern application development, performance and responsiveness are paramount.
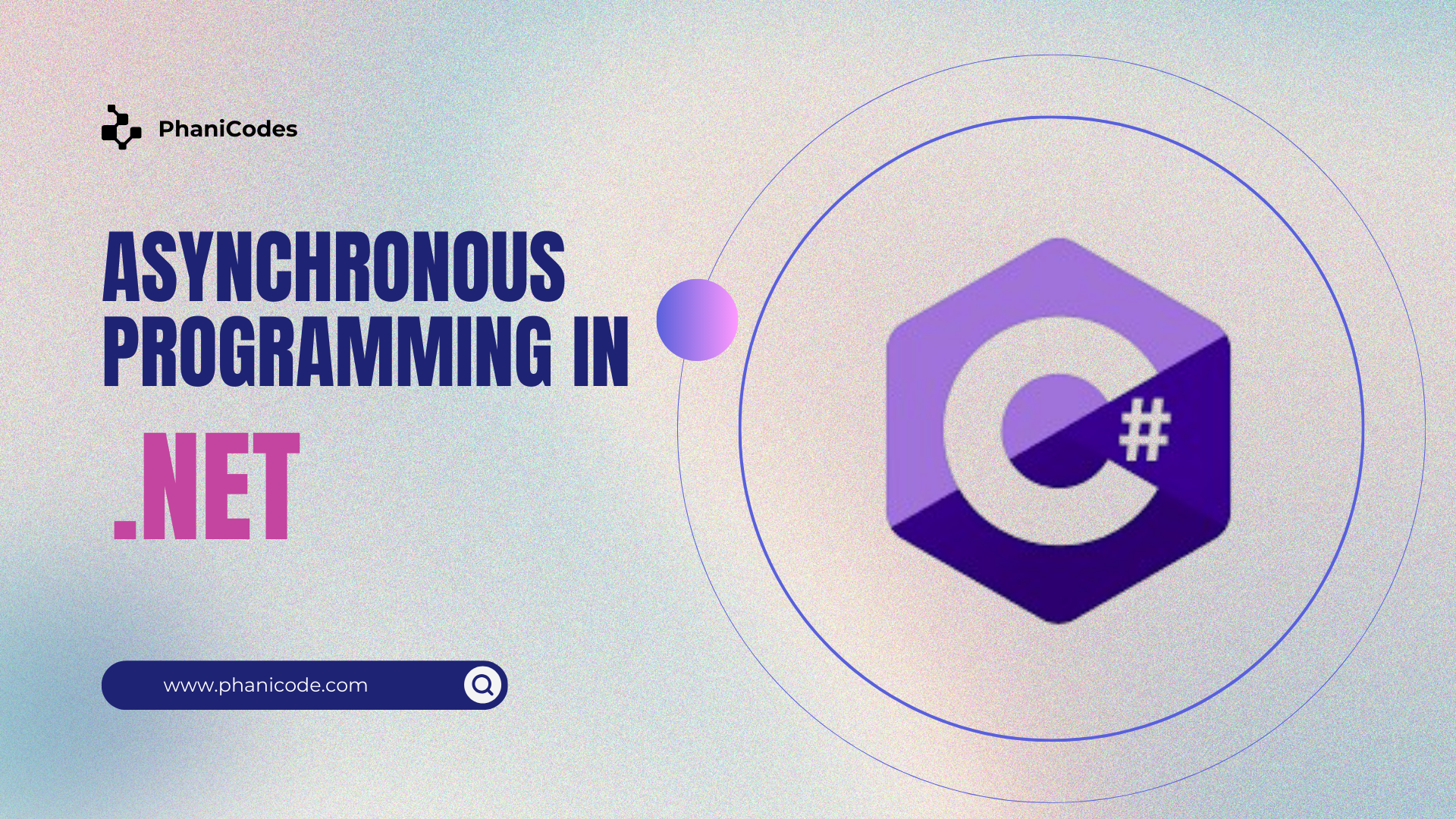
Introduction
In the realm of modern application development, performance and responsiveness are paramount. Synchronous operations, where the execution of a task blocks the main thread until it’s complete, can lead to sluggish user interfaces and reduced overall efficiency. Asynchronous programming offers a solution by allowing multiple tasks to execute concurrently, freeing up resources for other operations. This blog post will delve into the fundamental concepts of asynchronous programming in .NET, focusing on Tasks
and the async/await
keywords.
Understanding Tasks
A Task
in .NET represents an asynchronous operation. It encapsulates the execution of a method or block of code, allowing it to run independently on a different thread. When you create a Task
, you can start its execution and then await its completion, ensuring that your main thread doesn’t get blocked.
Creating and Starting a Task
Task task = Task.Run(() => {
// Code to be executed asynchronously
Console.WriteLine("Task is running...");
Thread.Sleep(2000);
Console.WriteLine("Task completed.");
});
Awaiting Task Completion
task.Wait(); // Blocks the current thread until the task completes
Using async/await
The async/await
keywords provide a more elegant and readable way to work with asynchronous operations. An async
method can contain await
expressions, which pause the execution of the method until the awaited task completes.
Example:
async Task DoWorkAsync()
{
Console.WriteLine("Starting work...");
await Task.Delay(2000);
Console.WriteLine("Work completed.");
}
async Task Main()
{
await DoWorkAsync();
}
Benefits of Async/Await
- Improved Readability:
async/await
makes asynchronous code look more like synchronous code, enhancing its readability. - Simplified Error Handling: Exceptions thrown within asynchronous methods can be handled using standard
try/catch
blocks. - Better Performance: By avoiding blocking operations, asynchronous code can improve application responsiveness and throughput.
Best Practices
- Use
async
for Methods That Perform I/O Operations: Methods that involve network calls, database queries, or file operations are good candidates for asynchronous programming. - Avoid Blocking Operations Within
async
Methods: Be cautious of synchronous operations withinasync
methods as they can defeat the purpose of asynchronous programming. - Consider Task Parallel Library (TPL) for CPU-Bound Tasks: For tasks that are computationally intensive, the TPL can provide efficient parallel execution.
Conclusion
Asynchronous programming is a powerful tool for building responsive and efficient .NET applications. By understanding the concepts of Tasks
and async/await
, you can effectively leverage these features to improve the user experience and overall performance of your software.