· Phani Veludurthi · Dotnet · 2 min read
Exploring .NET Dependency Injection: A Practical Guide
Dependency Injection (DI) is a software design pattern that promotes loose coupling between components
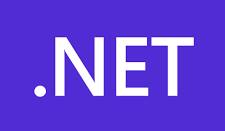
Introduction
Dependency Injection (DI) is a software design pattern that promotes loose coupling between components, making your code more maintainable, testable, and reusable. In .NET, DI is a core principle of the .NET Core framework.
Benefits of Dependency Injection
- Improved Testability: DI makes it easier to write unit tests by isolating components and providing mock implementations.
- Enhanced Maintainability: Loose coupling reduces the impact of changes, making your codebase more resilient to modifications.
- Increased Reusability: Components can be reused in different contexts without tight dependencies.
- Better Code Organization: DI encourages a more modular and organized architecture.
Implementing Dependency Injection in .NET Projects
1. Service Registration:
- Define interfaces to represent services.
- Create concrete implementations of these interfaces.
- Register the services with a DI container.
public interface IGreetingService
{
string Greet(string name);
}
public class GreetingService : IGreetingService
{
public string Greet(string name) => $"Hello, {name}!";
}
public class Startup
{
public void ConfigureServices(IServiceCollection services)
{
services.AddScoped<IGgreetingService, GreetingService>();
}
}
2. Dependency Injection:
- Inject dependencies into classes using constructor injection.
public class GreetingController
{
private readonly IGreetingService _greetingService;
public GreetingController(IGreetingService greetingService)
{
_greetingService = greetingService;
}
public IActionResult Greet(string name)
{
var greeting = _greetingService.Greet(name);
return Content(greeting);
}
}
3. Lifetime Management:
- Choose the appropriate lifetime for your services:
- Singleton: One instance per application.
- Scoped: One instance per HTTP request.
- Transient: A new instance for each request.
services.AddSingleton<IGreetingService, GreetingService>(); // Singleton
services.AddScoped<IGreetingService, GreetingService>(); // Scoped
services.AddTransient<IGreetingService, GreetingService>(); // Transient
Best Practices for Dependency Injection
- Prefer constructor injection: It’s more explicit and easier to manage dependencies.
- Avoid circular dependencies: Ensure a clear dependency graph.
- Use interfaces for abstractions: This promotes loose coupling.
- Consider using a DI framework: .NET Core includes a built-in DI container, but you can also explore third-party options like Autofac or Simple Injector.
Conclusion
Dependency Injection is a powerful tool for building well-structured and maintainable .NET applications. By understanding its principles and best practices, you can significantly improve the quality and flexibility of your code.