· Phani Veludurthi · .NET · 3 min read
Mastering LINQ: Querying Data with C#
LINQ (Language-Integrated Query) is a powerful feature in C# that provides a unified way to query data from various sources, including in-memory collections, databases, XML documents, and more. By leveraging LINQ, developers can write
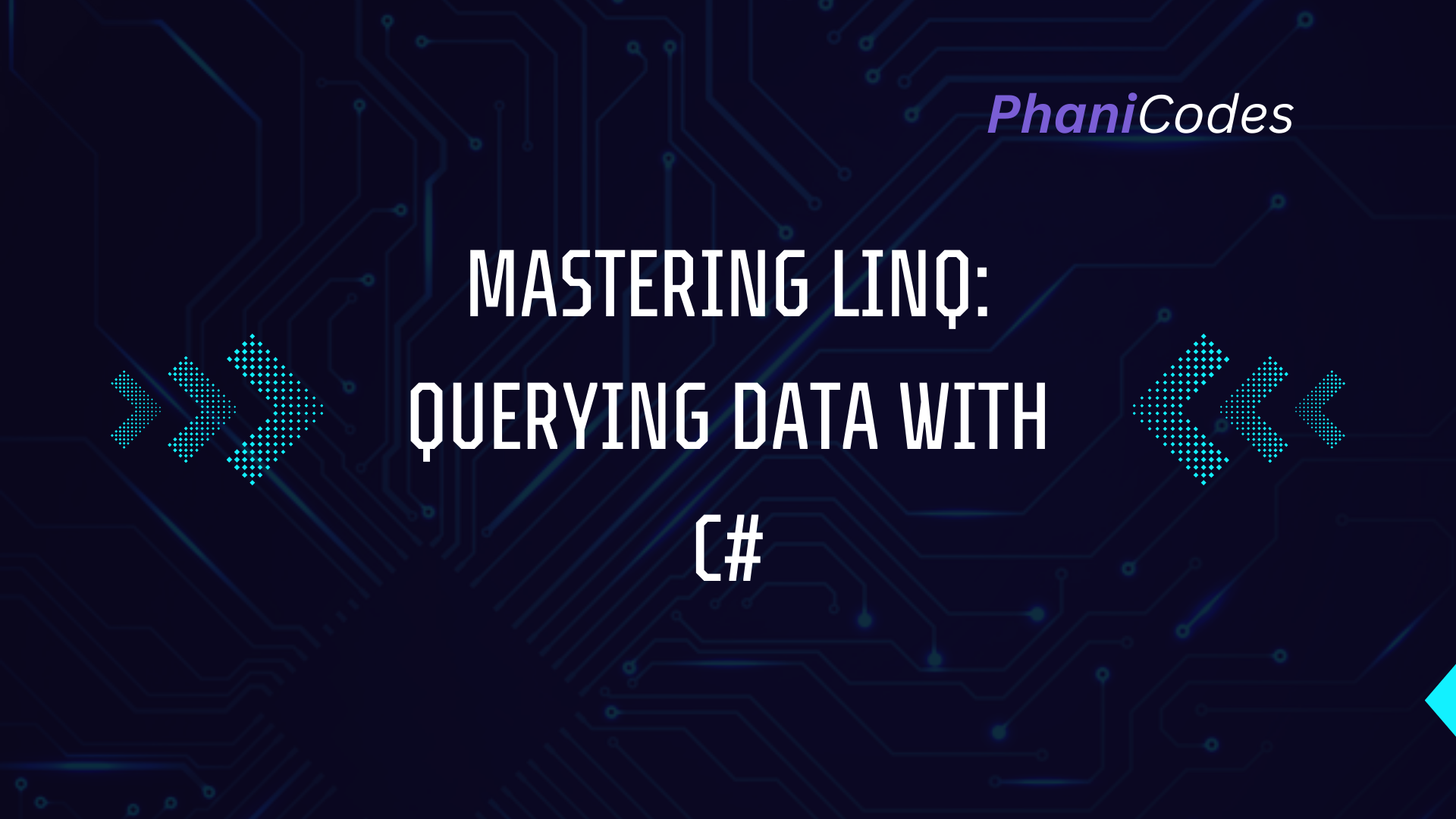
Introduction
LINQ (Language-Integrated Query) is a powerful feature in C# that provides a unified way to query data from various sources, including in-memory collections, databases, XML documents, and more. By leveraging LINQ, developers can write expressive and concise code to retrieve, filter, and transform data.
In this blog post, we’ll delve into the capabilities of LINQ and explore practical examples of how to use it to query data efficiently.
What is LINQ?
LINQ, or Language-Integrated Query, is a powerful feature in C# that allows you to query data from various sources in a unified way. It provides a declarative syntax that makes it easier to express your data manipulation intentions.
LINQ Query Syntax
The query syntax is a more readable and expressive way to write LINQ queries. It uses a syntax similar to SQL, making it familiar to developers who are already comfortable with SQL.
var query = from customer in customers
where customer.Age > 30
select customer;
LINQ Method Syntax
The method syntax is another way to write LINQ queries. It uses method calls on the data source to perform the query operations.
var query = customers.Where(customer => customer.Age > 30);
Common LINQ Operators:
- Filtering Operators:
Where
: Filters elements based on a condition.OfType
: Filters elements of a specific type.
- Projection Operators:
Select
: Projects elements to a new form.SelectMany
: Projects each element of a collection to anIEnumerable<T>
and flattens the resulting collections.
- Ordering Operators:
OrderBy
: Sorts elements in ascending order.OrderByDescending
: Sorts elements in descending order.
- Grouping Operators:
GroupBy
: Groups elements by a key.
- Aggregate Operators:
Sum
: Calculates the sum of elements.Average
: Calculates the average of elements.Count
: Counts the number of elements.
Querying In-Memory Collections
LINQ can be used to query in-memory collections like lists, arrays, and dictionaries.
var numbers = new List<int> { 1, 2, 3, 4, 5 };
var evenNumbers = numbers.Where(n => n % 2 == 0);
Querying Databases with LINQ to SQL
LINQ to SQL allows you to query databases using LINQ. You need to define a mapping between your C# classes and database tables.
using (var db = new DataContext())
{
var customers = db.Customers.Where(c => c.Age > 30).ToList();
}
Querying XML with LINQ to XML
LINQ to XML provides a way to query and manipulate XML documents using LINQ.
var xDocument = XDocument.Load("customers.xml");
var customers = xDocument.Descendants("customer").ToList();
Best Practices for LINQ
- Use the appropriate syntax: Choose between query syntax and method syntax based on readability and maintainability.
- Avoid unnecessary query execution: Only execute queries when necessary to improve performance.
- Use deferred execution: LINQ queries are deferred, meaning they are not executed until you iterate over the results.
- Consider performance implications: Be mindful of the performance impact of complex LINQ queries, especially when dealing with large datasets.
Conclusion
LINQ is a powerful tool for querying data in C#. By understanding its capabilities and best practices, you can write efficient and expressive code to manipulate data from various sources.